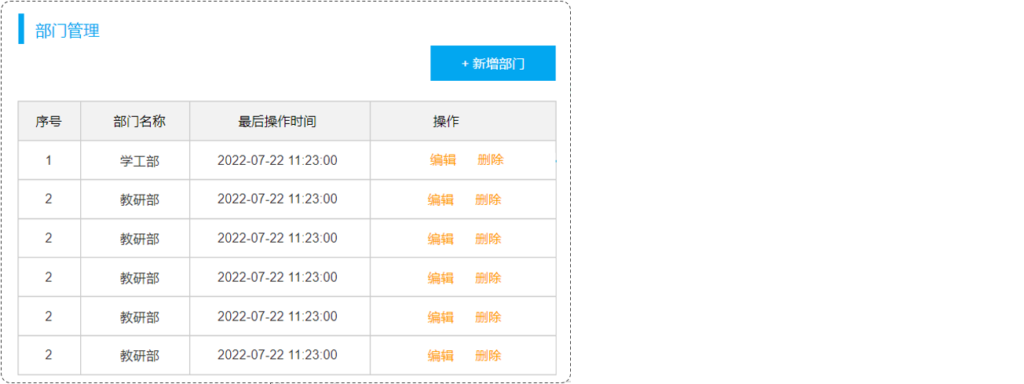
以部门管理为例,开发包括:
- 查询部门列表
- 删除部门
- 新增部门
- 修改部门(包括数据回显)
环境搭建
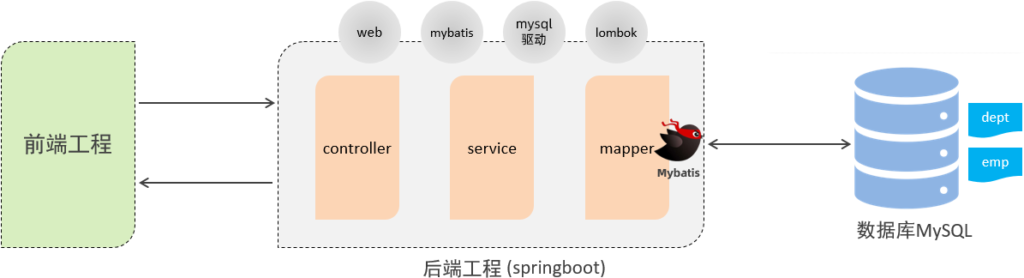
步骤:
- 准备数据库表(dept、emp)
- 创建springboot工程,引入对应的起步依赖(web、mybatis、mysql驱动、lombok)
- 配置文件application.properties中引入mybatis的配置信息,准备对应的实体类
- 准备对应的Mapper、Service(接口、实现类)、Controller基础结构
数据库表:
-- 部门管理
create table dept(
id int unsigned primary key auto_increment comment '主键ID',
name varchar(10) not null unique comment '部门名称',
create_time datetime not null comment '创建时间',
update_time datetime not null comment '修改时间'
) comment '部门表';
-- 部门表测试数据
insert into dept (id, name, create_time, update_time) values(1,'学工部',now(),now()),(2,'教研部',now(),now()),(3,'咨询部',now(),now()), (4,'就业部',now(),now()),(5,'人事部',now(),now());
创建项目工程目录结构:
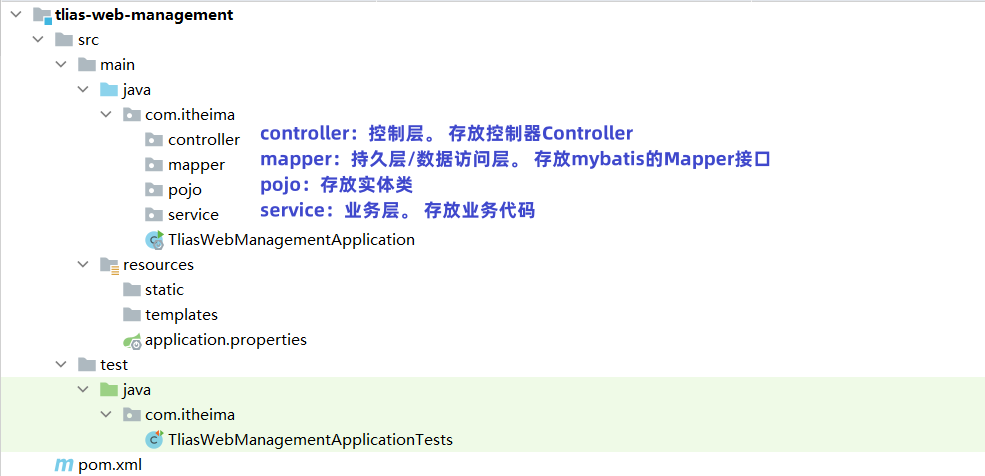
配置application.properties
#数据库连接
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/tlias
spring.datasource.username=root
spring.datasource.password=123456
#开启mybatis的日志输出
mybatis.configuration.log-impl=org.apache.ibatis.logging.stdout.StdOutImpl
#开启数据库表字段 到 实体类属性的驼峰映射
mybatis.configuration.map-underscore-to-camel-case=true
实体类:
@Data
@NoArgsConstructor
@AllArgsConstructor
public class Dept {
private Integer id; //部门id
private String name; //部门名称
private LocalDateTime createTime; //部门创建时间
private LocalDateTime updateTime; //部门信息修改时间
}
接口文档:
注:接口文档中的相应数据样例,只需关注data中数据,来确定对应返回值。
持久层
持久层配合Mybatis提供的注解进行编写。除查询有对应返回值外,增删改均无需返回值。
DeptMapper
@Mapper
public interface DeptMapper {
// 查询所有部门
@Select("select * from dept")
List<Dept> list();
// 根据id删除部门
@Delete("delete from dept where id = #{id}")
void delete(Integer id);
// 添加部门
@Insert("insert into dept (name, create_time, update_time) VALUES (#{name}, #{createTime}, #{updateTime})")
void insert(Dept dept);
// 根据id查询部门,用于数据回显
@Select("select * from dept where id = #{id}")
Dept selectById(Integer id);
// 根据id更新部门
@Update("update dept set name=#{name}, update_time=#{updateTime} where id=#{id}")
void update(Dept dept);
}
业务层
为了降低代码耦合性,Service分为接口和实现类。需要注意的是,接口不添加@Service注解,而添加在实现类上。
DeptService
public interface DeptService {
/**
* @description 查询所有部门
* @return List<Dept>
* @author fangkun
* @date 2023/10/11 20:43
**/
List<Dept> list();
/**
* @description 根据id删除部门
* @param id 入参
* @return void
* @author fangkun
* @date 2023/10/11 21:04
**/
void delete(Integer id);
/**
* @description 添加部门
* @param dept 入参
* @return void
* @author fangkun
* @date 2023/10/11 21:10
**/
void add(Dept dept);
/**
* @description 根据id查询部门
* @param id 入参
* @return Dept
* @author fangkun
* @date 2023/10/11 21:18
**/
Dept selectById(Integer id);
/**
* @description 根据id更新部门
* @param dept 入参
* @return void
* @author fangkun
* @date 2023/10/11 21:26
**/
void update(Dept dept);
}
DeptServiceImpl
@Slf4j //日志,简化代码
@Service
public class DeptServiceImpl implements DeptService {
@Autowired
public DeptMapper deptMapper;
@Override
public List<Dept> list() {
List<Dept> deptList = deptMapper.list();
return deptList;
}
@Override
public void delete(Integer id) {
deptMapper.delete(id);
}
@Override
public void add(Dept dept) {
// 创建时间和修改时间在业务层修改
dept.setCreateTime(LocalDateTime.now());
dept.setUpdateTime(LocalDateTime.now());
deptMapper.insert(dept);
}
@Override
public Dept selectById(Integer id) {
Dept dept = deptMapper.selectById(id);
return dept;
}
@Override
public void update(Dept dept) {
dept.setUpdateTime(LocalDateTime.now());
deptMapper.update(dept);
}
}
控制层
Controller层,我们使用Restful规范来进行编写。
DeptController
@Slf4j
@RestController
@RequestMapping("/depts")
public class DeptController {
@Autowired
private DeptService deptService;
@GetMapping
public List<Dept> list(){
log.info("查询部门");
List<Dept> deptList = deptService.list();
return deptList;
}
@DeleteMapping("/{id}")
public void delete(@PathVariable Integer id){
log.info("根据id删除部门");
deptService.delete(id);
}
@PostMapping
public void add(@RequestBody Dept dept){
log.info("添加部门");
deptService.add(dept);
}
@GetMapping("/{id}")
public Dept selectById(@PathVariable Integer id){
log.info("根据id查询部门");
Dept dept = deptService.selectById(id);
return dept;
}
@PutMapping
public void update(@RequestBody Dept dept){
log.info("根据id更新部门");
deptService.update(dept);
}
}